When working with image segmentation in Python, particularly with labeled regions, it’s common to use the label2rgb
function from the scikit-image
library to create colorful visualizations. This function is extremely helpful in mapping labeled regions to a visually distinctive RGB representation. However, a frequent question developers face is: how do we display a legend that clearly shows which color corresponds to which label?
Unfortunately, label2rgb
does not include built-in support to generate or display legends. But don’t worry — with some additional code and the help of Matplotlib, we can easily create and display a legend that aligns with the labels and colors shown in the label2rgb
output.
What Does label2rgb Do?
The label2rgb
function replaces labels in a 2D (or 3D) array with unique RGB colors, enabling better visualization of labeled regions. Each integer in the labeled image corresponds to a region, and label2rgb
assigns a color to it.
Here’s a simple example:
from skimage.color import label2rgb
from skimage.measure import label
from skimage import data
import matplotlib.pyplot as plt
image = data.coins()
binary = image > 100
labeled = label(binary)
colored_image = label2rgb(labeled, image=image, bg_label=0)
plt.imshow(colored_image)
plt.axis('off')
plt.show()
This renders perfectly, but without a legend, the viewer has no idea what each colored region represents. So let’s see how to add context.
Steps to Add a Legend
You can manually create a legend by retrieving the unique labels and assigning colors using a colormap. Here’s how:
- Extract Unique Labels: Use
np.unique()
to find all unique labels in the labeled image. - Generate Color Map: Get a consistent color mapping used by
label2rgb
. - Create Custom Patches: Use Matplotlib’s
Patch
to build legend entries.
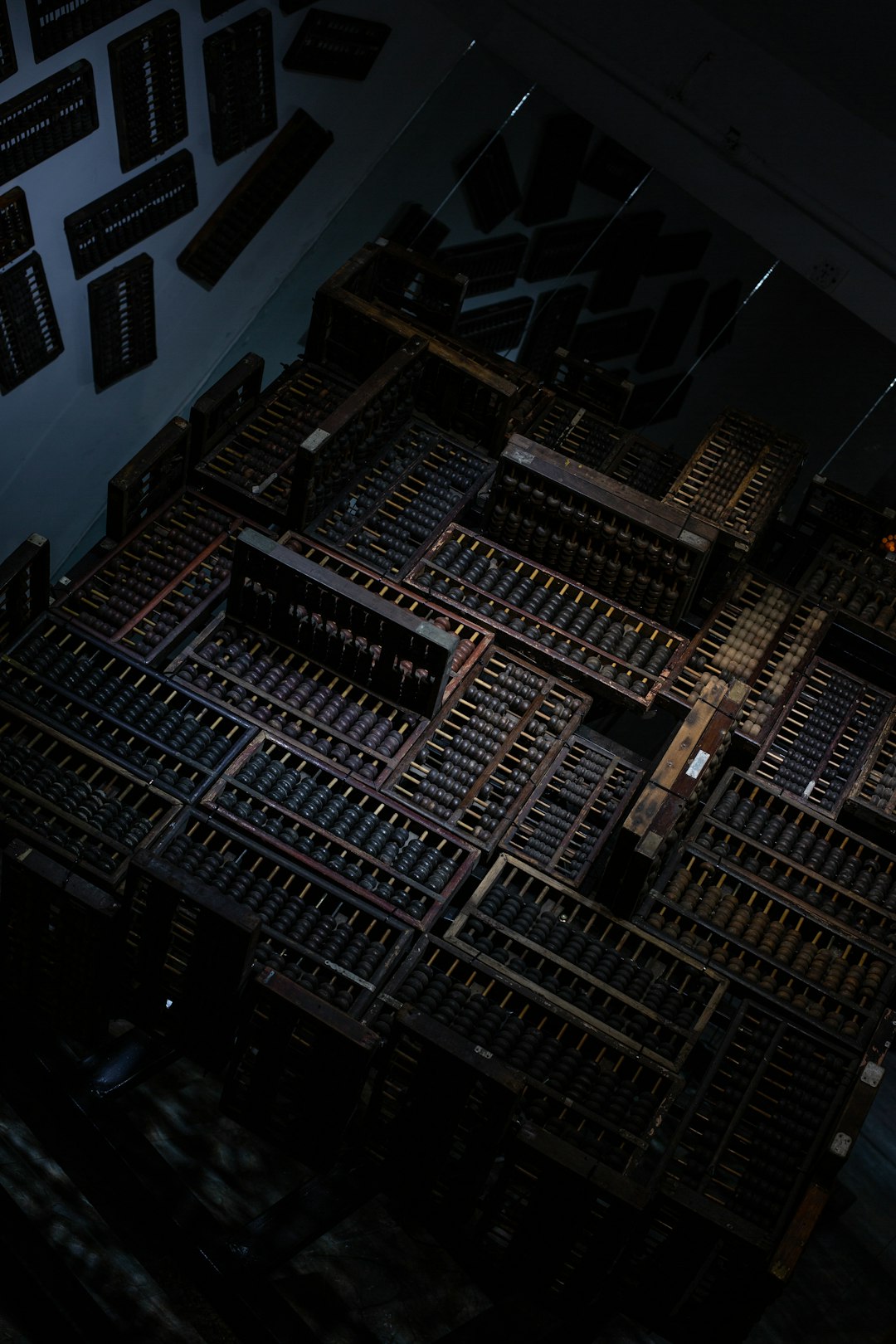
Here’s a more complete example with a legend:
import numpy as np
from skimage.data import coins
from skimage.filters import threshold_otsu
from skimage.measure import label
from skimage.color import label2rgb
import matplotlib.pyplot as plt
from matplotlib.patches import Patch
from matplotlib import cm
# Load and process the image
image = coins()
thresh = threshold_otsu(image)
binary = image > thresh
labeled_image = label(binary)
# Generate RGB image
colored_image = label2rgb(labeled_image, image=image, bg_label=0)
# Get unique labels
unique_labels = np.unique(labeled_image)
unique_labels = unique_labels[unique_labels != 0] # Exclude background
# Assign colors
cmap = cm.get_cmap('nipy_spectral', len(unique_labels))
legend_handles = []
for idx, label_val in enumerate(unique_labels):
color = cmap(idx) # Get RGBA tuple
label_name = f'Region {label_val}'
patch = Patch(color=color, label=label_name)
legend_handles.append(patch)
# Display image with legend
plt.figure(figsize=(10, 6))
plt.imshow(colored_image)
plt.axis('off')
plt.legend(handles=legend_handles, bbox_to_anchor=(1.05, 1), loc='upper left')
plt.tight_layout()
plt.show()
Tips for Better Visualizations
- Use descriptive names: If the labels correspond to real-world objects (e.g., cells, coins, people), rename them for clarity.
- Control color randomness: Set the
colors
argument inlabel2rgb
for consistent coloring between runs. - Use colormaps wisely: Select perceptually uniform colormaps from Matplotlib to aid in accessibility and clarity.
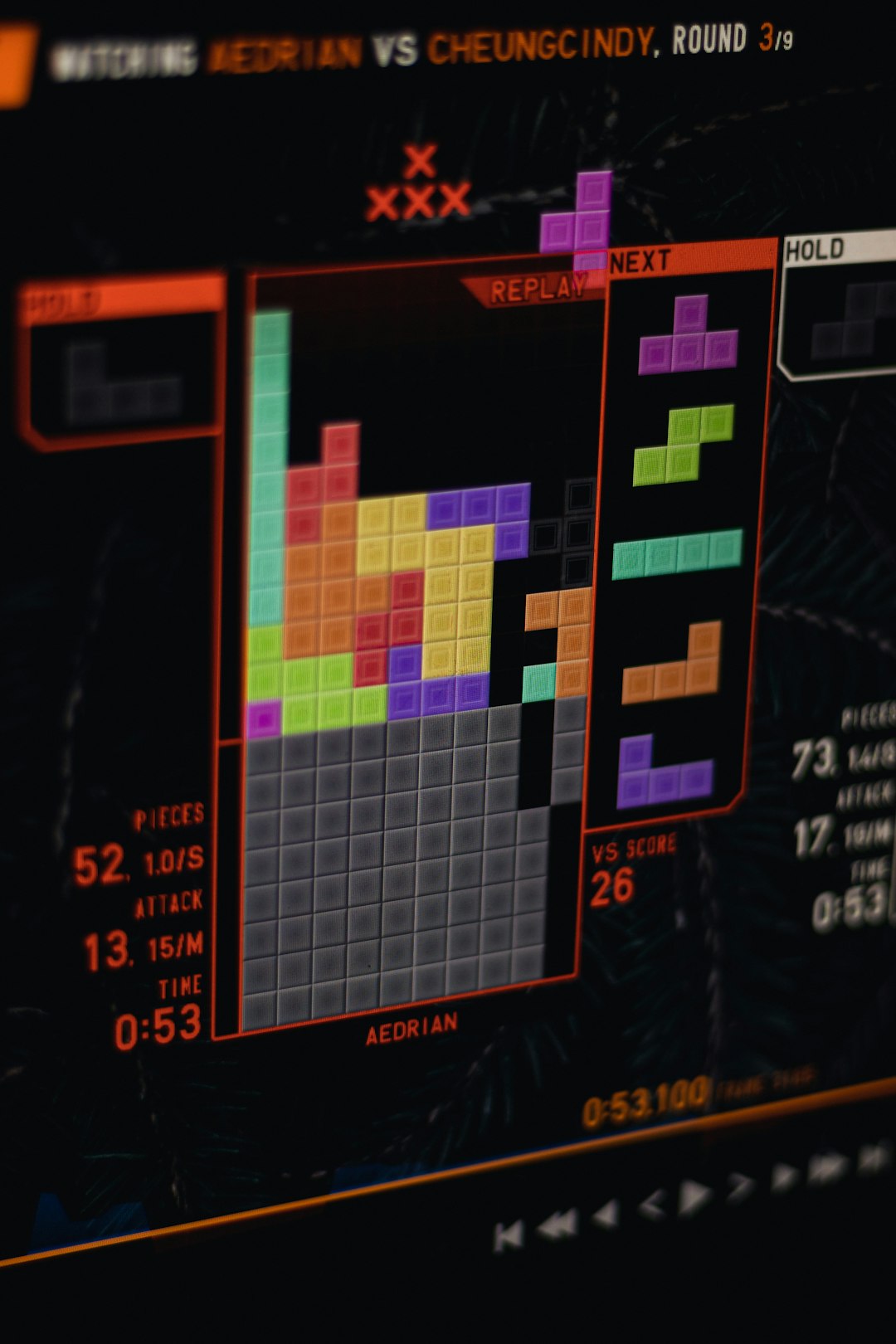
Why is the Legend Important?
In image analysis, particularly in fields like medical imaging or satellite data, interpretable visualizations are crucial. A legend transforms raw, classified regions into meaningful data by helping users understand which areas correspond to specific classes or labels.
Conclusion
Although label2rgb
simplifies the task of visualizing labeled regions in an image, it leaves room for customization when it comes to interpretability. Thankfully, with just a bit of extra code and help from Matplotlib legends and patches, you can significantly enhance the communicative power of your visualizations.
So next time you’re presenting segmented results, whether for a report or a scientific paper, consider adding a legend — your viewers will thank you!