In the world of Java programming, one of the most frequently encountered commands is System.out.print. For beginners and even for some intermediate developers, understanding what this line of code does and why it’s used is essential for writing functional and interactive applications. This command is neither complex nor trivial — it is a tool that gives developers a way to communicate with users via the console. Knowing how it works is the first step in mastering basic Java I/O operations.
The System.out.print statement is part of Java’s standard output mechanism. To make sense of it, it’s helpful to break it down into its components:
- System – This is a built-in Java class contained in the java.lang package. It provides access to system-level properties and resources.
- out – This is a static member (specifically, a public static final field) of the System class. It is an instance of PrintStream, which represents the standard output stream.
- print – This is the method invoked on the out object. It outputs text to the console, without a newline character at the end.
This combination — System.out.print — effectively tells the Java Virtual Machine (JVM) to send text to the console for the user to read. When executed, the method displays the given string or value directly in the terminal or console window.
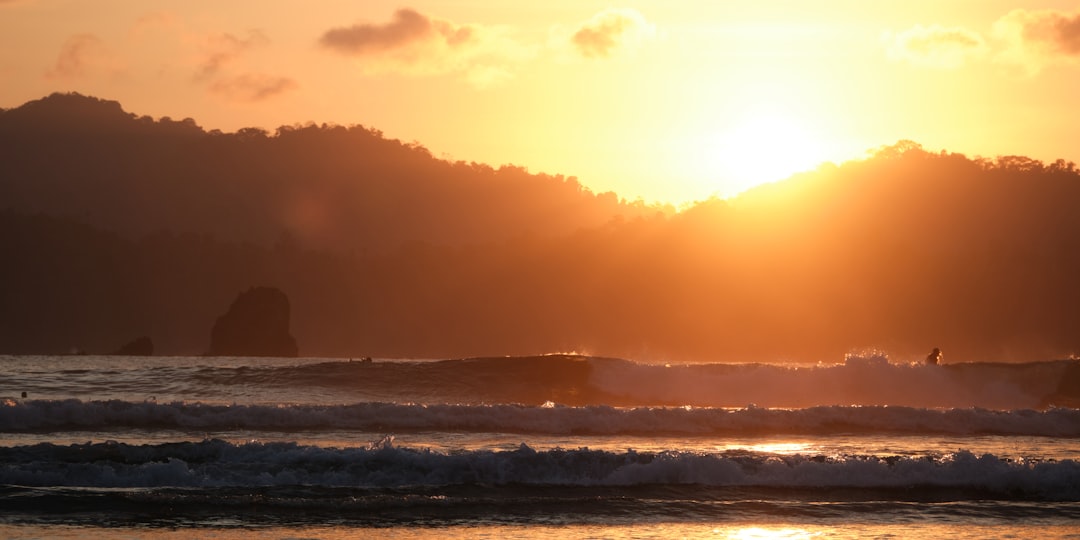
It’s important to note that System.out.print differs from System.out.println. While print simply writes text to the output, println additionally adds a new line, so the text printed after it starts on a new line. This small difference plays a large role in formatting program output properly.
Here is a simple example to illustrate the difference:
System.out.print("Hello, ");
System.out.print("world!");
System.out.println();
System.out.println("Welcome to Java.");
The output will be:
Hello, world!
Welcome to Java.
This example shows how print and println can be combined to control how information is displayed during execution.
Why Use System.out.print?
Though more advanced applications may use graphical or networked outputs, System.out.print still has several important use cases:
- Debugging: The simplest way to check the values of variables or execution flow is to print relevant information to the console.
- Educational purposes: Beginners often use the console as a training ground to understand fundamental code behavior.
- Simple applications: Command-line tools and utilities often rely solely on standard output and input.
For instance, developers learning how loops and conditionals work might use this method to print the current value of a variable during each iteration.
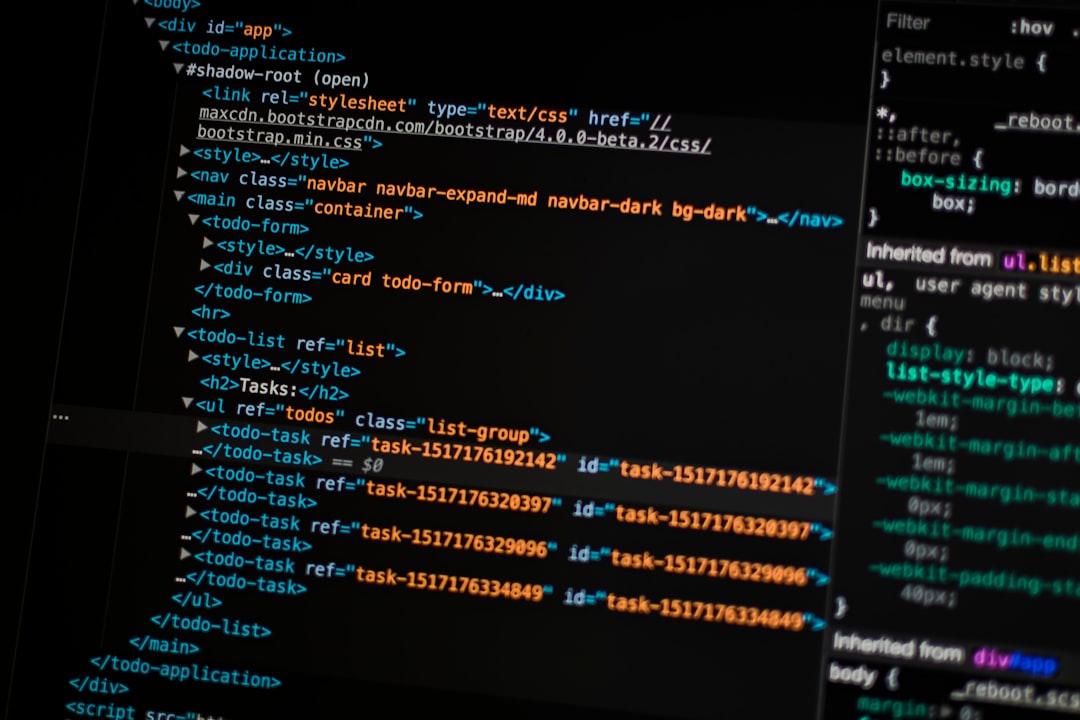
Technical Perspective: What Happens Behind the Scenes?
When System.out is called, it refers to a default PrintStream instance that is connected to the console by the JVM. The print() and println() methods in PrintStream are overloaded, meaning they can handle different data types like strings, integers, characters, and more. This allows Java developers to easily output a variety of data types without complex conversions or formatting.
Additionally, output to the console is usually buffered, meaning that the text might be temporarily stored before being displayed, depending on how the application is executed and the environment it runs in.
Best Practices and Considerations
While System.out.print is a valuable tool, there are a few best practices worth noting:
- In production-level code, avoid using standard output for logging. Instead, use a dedicated logging framework like Log4j or SLF4J for flexibility and control.
- Format your output cleanly to make it intuitive for users or developers analyzing it.
- Remember that standard output might be redirected in some environments, such as servers or automated testing frameworks, so don’t rely on it for tracking mission-critical behaviors.
By adopting these practices, developers can maintain code quality while taking advantage of console output during the development and testing phases.
In conclusion, System.out.print represents an essential building block in Java programming. It provides a straightforward way to interact with users and inspect program behavior. Though basic, it holds significant value, especially in learning and debugging contexts. Understanding its structure and purpose forms a foundational skill for any aspiring Java developer.