In the realm of enterprise software development, maintaining a record of data changes is an essential requirement for many applications. Whether for compliance, debugging, or security, tracking who changed what and when can prove critical. Spring Data JPA offers a robust and flexible auditing feature built-in, enabling developers to keep track of entity changes with minimal configuration and code.
What Is Auditing in Spring Data JPA?
Auditing refers to the automatic capturing of information such as creation and update timestamps, along with user identifiers associated with creating and modifying entities. This capability helps developers record lifecycle events of persistent entities without writing extra logging or tracking code.
With Spring Data JPA, auditing becomes remarkably straightforward thanks to its integration with the Spring Framework and Hibernate. By simply using annotations and enabling specific configurations, the framework takes care of most of the heavy lifting.
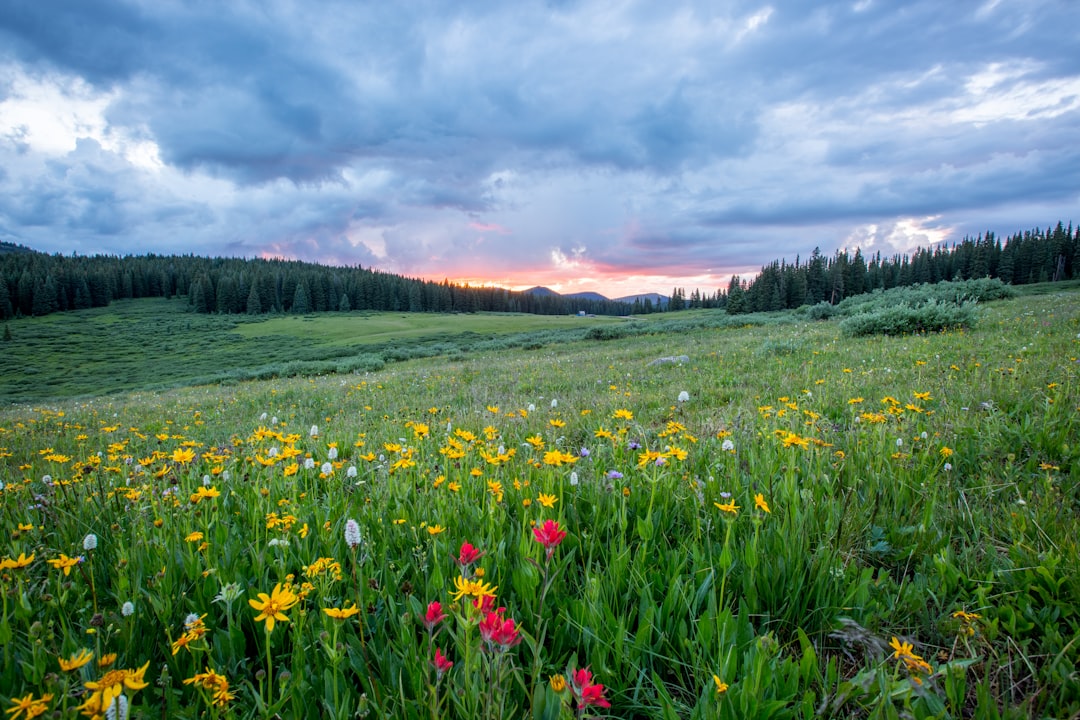
Key Annotations for Auditing
Spring Data JPA provides four main annotations for entity auditing:
- @CreatedDate – Captures the timestamp when the entity was created.
- @LastModifiedDate – Captures the timestamp when the entity was last updated.
- @CreatedBy – Stores the identifier of the user who created the entity.
- @LastModifiedBy – Stores the identifier of the user who last updated the entity.
These annotations are used within JPA entity classes, typically in combination with a base class shared across multiple entities.
Setting Up Spring Data JPA Auditing
To enable auditing in a Spring Boot project that uses Spring Data JPA, follow these steps:
1. Enable JPA Auditing
In your configuration class, add the @EnableJpaAuditing
annotation. Optionally, you can specify a reference to an auditor-aware bean.
@Configuration
@EnableJpaAuditing(auditorAwareRef = "auditorProvider")
public class PersistenceConfig {
}
2. Create AuditorAware Bean
This bean supplies the current auditor (usually the username or user ID). It returns an Optional<String>
or similar object based on the application’s authentication context.
@Bean
public AuditorAware<String> auditorProvider() {
return () -> Optional.of(SecurityContextHolder.getContext().getAuthentication().getName());
}
3. Add Auditing Fields in Your Entity
Use the auditing annotations in your entity class, along with the @EntityListeners(AuditingEntityListener.class)
annotation.
@Entity
@EntityListeners(AuditingEntityListener.class)
public class Product {
@Id
@GeneratedValue
private Long id;
@CreatedDate
private LocalDateTime createdDate;
@LastModifiedDate
private LocalDateTime modifiedDate;
@CreatedBy
private String createdBy;
@LastModifiedBy
private String modifiedBy;
// Getters and setters
}
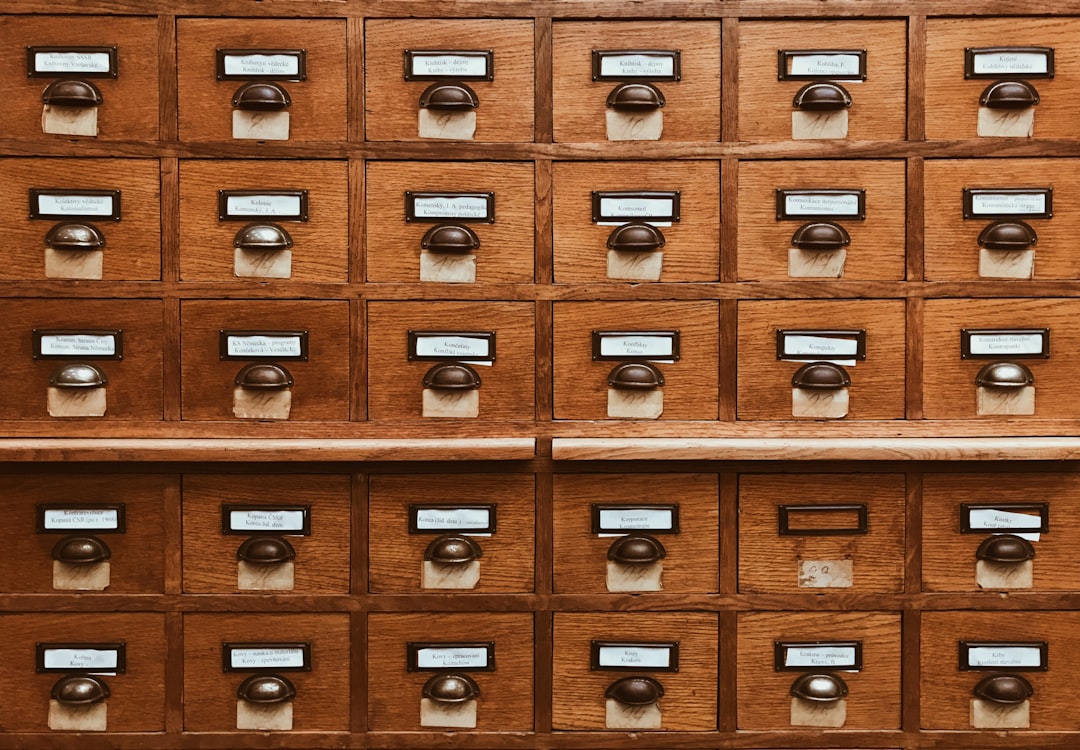
Use Cases for Entity Auditing
Auditing is widely used in several scenarios, including:
- Compliance and Audit Trails: Organizations in regulated industries use auditing to provide traceability of data operations.
- Security Event Logging: Identifying unauthorized or suspicious changes to data.
- Debugging: Tracking unexpected data changes in logs during software troubleshooting.
Advanced Considerations
While basic auditing is simple to configure, complex applications may require enhancements, such as:
- Using UUIDs or custom user identifiers for creators/modifiers.
- Auditing soft-deleted records or archived data.
- Storing audit logs in a separate table or even a NoSQL database for scalability.
For such cases, developers might consider using additional tools like Envers or writing custom event listeners.
Conclusion
Auditing with Spring Data JPA is both powerful and easy to implement. With just a few annotations and configurations, developers can automatically track entity lifecycle events, enabling better observability and compliance within their applications. Spring’s pluggable design also makes it easy to extend and adapt the auditing system to fit a wide range of business requirements.
FAQ
- Q1: Does Spring Data JPA auditing require a specific database?
- No. It works with any relational database supported by JPA/Hibernate, such as MySQL, PostgreSQL, and Oracle.
- Q2: Can I customize the auditor format, such as storing user IDs instead of names?
- Yes. The
AuditorAware
implementation can return any identifier (e.g., ID, username, custom token) as long as it matches the data type in your entity fields. - Q3: Is it possible to audit soft deletes using Spring Data JPA?
- Yes. You need to implement a custom auditing strategy or use tools like Hibernate Envers to track the soft delete status.
- Q4: Can I exclude certain entities from auditing?
- Yes. Simply do not annotate those entities with
@EntityListeners(AuditingEntityListener.class)
or omit field-level auditing annotations. - Q5: Is auditing thread-safe?
- Auditing is safe within the transaction context managed by Spring and Hibernate. However, ensure that your AuditorAware implementation is thread-safe.